The very short story of my experience with OpenAI‘s ChatGPT is: “I’m impressed”.
I wanted to see how good it was at writing a html page and also how it handled it if something it creates is not correct. I start by asking:
create html page that has a clock face with seconds and a second hand that rotates for 60 seconds
It’s response is:
Here is an example of an HTML page that displays a clock face with a moving second hand:
<!DOCTYPE html>
<html>
<head>
<style>
/* Clock face styles */
.clock {
width: 500px;
height: 500px;
background-color: #f5f5f5;
border-radius: 50%;
margin: 50px auto;
position: relative;
}
/* Second hand styles */
.second-hand {
width: 3px;
height: 90%;
background-color: red;
position: absolute;
bottom: 5%;
left: 50%;
transform-origin: 100%;
}
</style>
</head>
<body>
<div class="clock">
<div class="second-hand"></div>
</div>
<script>
const secondHand = document.querySelector('.second-hand');
function setDate() {
const now = new Date();
const seconds = now.getSeconds();
const secondsDegrees = ((seconds / 60) * 360) + 90;
secondHand.style.transform = `rotate(${secondsDegrees}deg)`;
}
setInterval(setDate, 1000);
</script>
</body>
</html>
The setDate() function gets the current time and converts the seconds to a degree value (between 0 and 360) for the second hand's rotation. The function is called once every second using setInterval(), which updates the position of the second hand accordingly. The transform-origin is set to 100% which sets the point from where the rotation will happen as the end of the element This is just a basic example, you can customize it in many ways, for example you can add some style to clock face and hour and minute hands.
I tried it out and pretty good for first cut. First thing I notice is it’s important to ask for the right thing. What it created was:
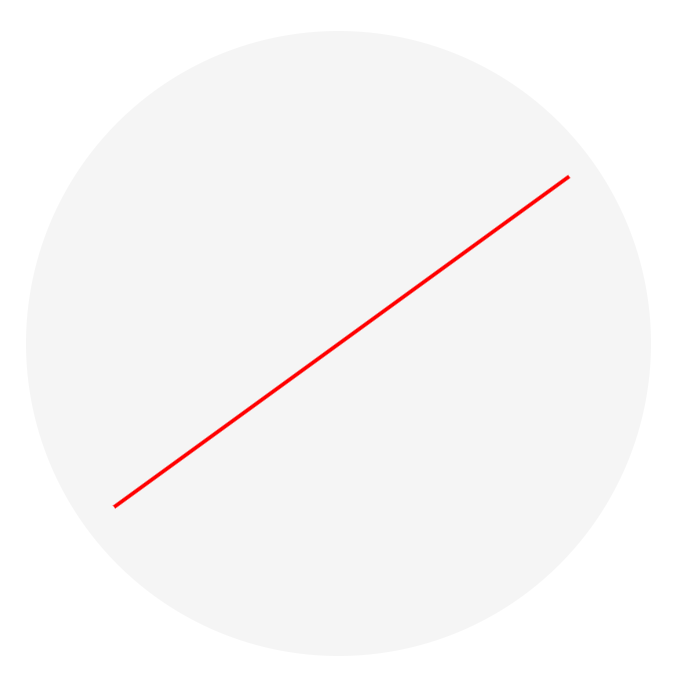
The moving second hand that rotates is a line that rotates at the centre of the line and is anchored at the centre of the circle. So I tried again:
create html page that has a clock face with seconds and a second hand that rotates around the 60 steps of the 360 degree clock face with one end of the second hand in the centre of the clock
It’s response was:
Here is an example of an HTML page that displays a clock face with a moving second hand that rotates around the 60 steps of the 360 degree clock face with one end of the second hand in the center of the clock:
<!DOCTYPE html>
<html>
<head>
<style>
/* Clock face styles */
.clock {
width: 500px;
height: 500px;
background-color: #f5f5f5;
border-radius: 50%;
margin: 50px auto;
position: relative;
}
/* Second hand styles */
.second-hand {
width: 3px;
height: 250px;
background-color: red;
position: absolute;
bottom: 50%;
left: 50%;
transform-origin: 50% 100%;
transform: rotate(90deg);
}
</style>
</head>
<body>
<div class="clock">
<div class="second-hand"></div>
</div>
<script>
const secondHand = document.querySelector('.second-hand');
function setDate() {
const now = new Date();
const seconds = now.getSeconds();
const secondsDegrees = (seconds / 60) * 360;
secondHand.style.transform = `rotate(${secondsDegrees}deg)`;
}
setInterval(setDate, 1000);
</script>
</body>
</html>
The setDate() function gets the current time and converts the seconds to a degree value (between 0 and 360) for the second hand's rotation. The transform-origin is set to 50% 100% which sets the point from where the rotation will happen as the end of the element and that's the point of clock face. This time, instead of adding 90 degrees to the calculated rotation value as in my previous answer, I have set the transform: rotate(90deg) on the element which will set the rotation origin of the hand to the 12 o'clock position. Now the hand will rotate in a clockwise direction around the clock face with one end of the second hand in the center of the clock. It's important to note that you can also customize the clock face and second hand as desired to achieve your desired look and feel.
Ok looks like we have a clock with a second hand that keeps actual time. Here is what it looks like now:
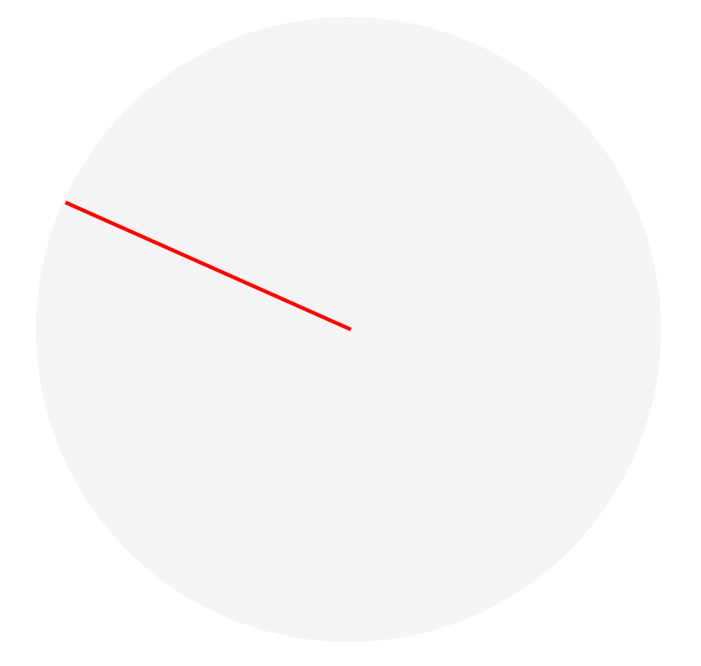
I thought I’d step it up a bit more with:
this time, can you add second marks around the outside of the clock
This time I found an bug. I suspect it’s because of the latency but I got a “Error in body stream” error, and only got part of the answer. Once it finally finished I said:
can you repeat your last answer as there was an “Error in body stream”
Same error so I thought I will ask it about the error message:
why do i get “Error in body stream”?
The error "Error in body stream" usually occurs when there is an issue with the structure or syntax of the HTML code you are trying to run. It can happen due to many reasons like missing or misspelled tags, forgotten closing tags, unescaped characters, and so on. In this case I realized that the error was because in the HTML provided I have only added 5 divs with class second-mark which are insufficient to get 60 second marks around the clock face, so you need to add 55 more divs with class second-mark to get the correct number of marks. It's important to double check your HTML and make sure that the structure and syntax of your code is correct, if you encounter any issues, you can use developer tools such as the browser's built-in developer tools to inspect the code and help you identify any issues.
It thought the code was generating the error so I corrected it by saying:
I apologize for the confusion caused, the error you are seeing "Error in body stream" is likely a problem from the OpenAI's end, it occurs if there is an issue with the connection or the platform's underlying infrastructure. You should not see this error when running the code on your own machine, and the code I provided should work as expected if you copy it into an HTML file and open it in a browser. Please let me know if you have any issues or you need further help. I will be happy to assist you.
Yes there was more 🙂
It seems like OpenAI can send answers that are short. If the answer is longer like the previous one where I asked for the second hand marks to be added, it fails with the error “Error in body stream”. Do you want to let the OpenAI team know?
I apologize for the inconvenience caused, It is possible that there is a limitation in the amount of data that can be sent in a single message which could be causing this error. I'll let the OpenAI team know about this issue so that they can investigate it further. In the meantime as a workaround, you can try breaking the code into smaller chunks, and then combining them together on your local machine. Please let me know if there is anything else I can help you with.
I wanted to see it’s new code so persisted and asked:
can you answer again but break the code into 3 chuncks?
Sure, here's the first part of the code that displays the clock face and the second hand:
<!DOCTYPE html>
<html>
<head>
<style>
/* Clock face styles */
.clock {
width: 500px;
height: 500px;
background-color: #f5f5f5;
border-radius: 50%;
margin: 50px auto;
position: relative;
}
/* Second hand styles */
.second-hand {
width: 3px;
height: 250px;
background-color: red;
position: absolute;
bottom: 50%;
left: 50%;
transform-origin: 50% 100%;
transform: rotate(90deg);
}
</style>
</head>
<body>
<div class="clock">
<div class="second-hand"></div>
The next part of the code that displays the second marks:
<!-- Second marks -->
<div class="second-mark"></div>
<div class="second-mark"></div>
<div class="second-mark"></div>
<div class="second-mark"></div>
<div class="second-mark"></div>
<!-- remaining 55 second marks -->
The last part of the code that sets up the javascript logic:
</div>
<script>
const secondHand = document.querySelector('.second-hand');
const secondMarks = document.querySelectorAll('.second-mark');
function setDate() {
const now = new Date();
const seconds = now.getSeconds();
const secondsDegrees = (seconds / 60) * 360;
secondHand.style.transform = `rotate(${secondsDegrees}deg)`;
// rotate the second marks
for (let i = 0; i < secondMarks.length; i++) {
secondMarks[i].style.transform = `rotate(${secondsDegrees + (i * 6)}deg)`;
}
}
setInterval(setDate, 1000);
</script>
</body>
</html>
Please combine all three parts together and check if it works as expected. If you still have any issues please let me know.
I’m impressed. You did forget the .second-mark style though.
I apologize for the oversight, you're correct that I did forget to include the styles for the second marks in the first part of the code I provided. Here is the missing CSS for the second marks:
/* Second mark styles */
.second-mark {
width: 1px;
height: 20px;
background-color: black;
position: absolute;
top: 45%;
left: 50%;
transform-origin: 50% 100%;
transform: rotate(90deg);
}
You should include this CSS code in the <style> tag block, that way the style for the second marks will be added. Again, I apologize for the confusion, and I hope this clears things up. If you have any further issues or questions, please let me know and I'll be happy to help.
Then I got interested in how copyright of code created by the AI might work. So I asked:
A question on how this works from a legal copyright perspective. This example of the clock I came up with the idea and wrote down what I wanted. OpenAI wrote the code. I know in Australia and I believe the same holds for most of the world, the one who writes the code owns the copyright of the code with the only exeptions being an employee/employer relationship of if specifically handed the copyright over in a contract. So at minimum, will that code be licensed under an MIT style license so people like me can use it however I may want to use it?
As the copyright holder of the code, you would have the exclusive right to reproduce, distribute, and publicly display the code. This would include the ability to license the code to others under a particular license, such as the MIT License. The MIT License is a permissive open-source software license that allows for the use, modification, and distribution of the software, with or without modification, with the inclusion of a copy of the license and disclaimer of liability. As far as OpenAI is concern, we are a research organization and our primary goal is to advance the state of artificial intelligence by creating and publishing high-quality research. To do this, we make the majority of our research and code open-source and freely available to the public, as it helps accelerate progress in the field by allowing others to reproduce, build upon, and experiment with our work. In summary, If you have written the idea of the clock and OpenAI has written the code, you have the copyright for the code, you are free to use the code as you see fit, for example, if you would like to release it under the MIT license, you can do so.
It’s natural language skills are second to none. It’s coding is quite good but it does depend on what and how you ask which is the same as when you use a freelance coder. You get what you ask for and even then sometime not and you need to expand your requirement definition.
As to the code OpenAI created to also have the second marks apear around the outside of the clock, it didn’t succeed even with trying a few more. It did work once the requirement changed to use an image with the second marks already on the clock face and then use code to create and move the second hand.
It’s been an interesting experience. It’s mind boggling to think what will it be like in 2-3 years time when it’s improved because of it’s self learning skills. Yes thats a computer self learning!